Lettuce first setup a Descriptive Flexfield with some segments before rendering it on the Self Service page. If you already know how to do this and are just anxious to see the few lines of code to reach the solution, scroll down in this post until you find it. For our example we will use the "Change Pay:
Pay Details" page in Manager Self Service and the "Add'l Salary Admin. Details" Descriptive Flexfield attached to it. Navigate as follows:
(N) System Administrator -> Application -> Flexfield -> Descriptive -> Segments
Search for "Add'l Salary Admin. Details" and do the following:
- Uncheck "Freeze Flexfield Definition"
- Highlight "Global Data Elements"
- Add a Context called 'Oracle Hack' for the Code and Name
- Click the Segments button
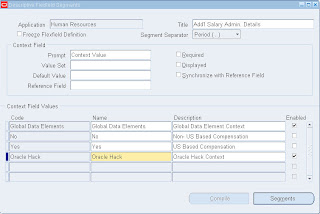
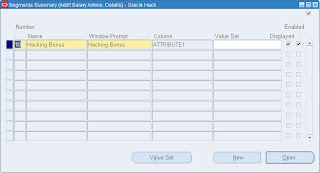
Uncheck the Required Checkbox:
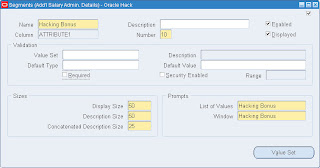
Save the DFF, Freeze Flexfield Definition and exit.
The first step to defaulting a DFF value on a Self Service page is to enable it. Browse to Manager Self Service -> Change Pay:
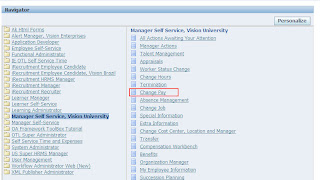
Choose an employee and click Action:
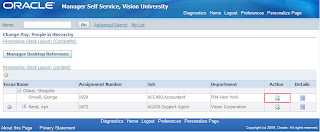
Click Propose Pay Change. On the "Change Pay: Pay Details" page, the DFF is now rendered under the Additional Details section:
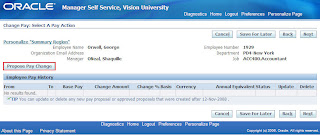
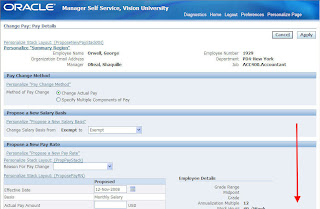
Choosing the "Oracle Hack" Context then displays the one segment defined for it:
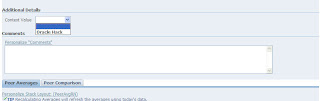
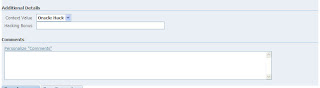
The goal of this exercise is to default the context as well as the segment value when the page loads. In order to do this, a Controller Class extension will need to be created that finds the DFF field and defaults the appropriate values. A cursory review of the "About this Page" link will show which CO's are already defined and where they are stored on the middle tier.
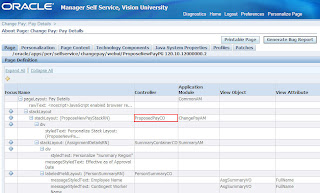
An extension to the oracle.apps.per.selfservice.changepay.webui.ProposedPayCO Controller Class will be performed in order to accomplish the defaulting:
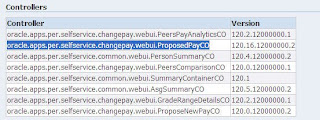
The remaining steps to perform the defaulting are as follows:
- Download the page definition of /oracle/apps/per/selfservice/changepay/webui/ProposeNewPayPG from the database using jdr_utils.printDocument
- Import the page into JDeveloper
- Set a new controller class for the page that extends the default CO oracle.apps.per.selfservice.changepay.webui.ProposedPayCO
- write custom code to find and default the DFF fields
As mentioned earlier, the detailed instructions for steps 1-3 are explained in previous posts. The code in step 4 is presented here:
/*===========================================================================+
| Copyright (c) 2001, 2005 Oracle Corporation, Redwood Shores, CA, USA |
| All rights reserved. |
+===========================================================================+
| HISTORY |
+===========================================================================*/
package hack.oracle.apps.per.selfservice.changepay.webui;
import oracle.apps.fnd.common.VersionInfo;
import oracle.apps.fnd.framework.webui.OAPageContext;
import oracle.apps.fnd.framework.webui.beans.OADescriptiveFlexBean;
import oracle.apps.fnd.framework.webui.beans.OAWebBean;
import oracle.apps.fnd.framework.webui.beans.message.OAMessageTextInputBean;
// import the delivered CO
import oracle.apps.per.selfservice.changepay.webui.ProposedPayCO;
/**
* Controller for ...
*/
public class hackProposedPayCO extends ProposedPayCO
{
public static final String RCS_ID="$Header$";
public static final boolean RCS_ID_RECORDED =
VersionInfo.recordClassVersion(RCS_ID, "%packagename%");
/**
* Layout and page setup logic for a region.
* @param pageContext the current OA page context
* @param webBean the web bean corresponding to the region
*/
public void processRequest(OAPageContext pageContext, OAWebBean webBean)
{
super.processRequest(pageContext, webBean);
// first, find the flexfield's handle
OADescriptiveFlexBean oaDFF = (OADescriptiveFlexBean)webBean.findIndexedChildRecursive("FlexField1");
// default the Attribute Category (DFF context)
oaDFF.setFlexContext(pageContext,"Oracle Hack");
oaDFF.processFlex(pageContext);
// now set the attribute/segment value
OAMessageTextInputBean txtHackBonus = (OAMessageTextInputBean)oaDFF.findIndexedChild("FlexField11");
txtHackBonus.setText("43500");
}
/**
* Procedure to handle form submissions for form elements in
* a region.
* @param pageContext the current OA page context
* @param webBean the web bean corresponding to the region
*/
public void processFormRequest(OAPageContext pageContext, OAWebBean webBean)
{
super.processFormRequest(pageContext, webBean);
}
}
Once you are done coding, do a "Make" and "Rebuild" of the file and transfer it to $JAVA_TOP on the middle tier. Browse to the "Change Pay: Pay Details" page in Manager Self Service and personalize it to point at your new Controller:
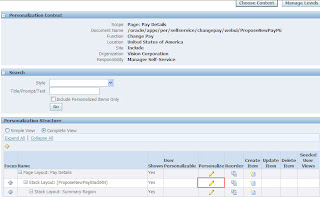
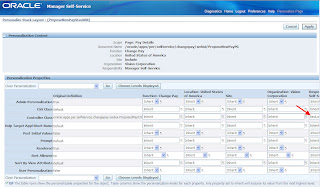
Set the value of the new Controller to: hack.oracle.apps.per.selfservice.changepay.webui.hackProposedPayCO
Return to the Application and marvel at your handiwork:
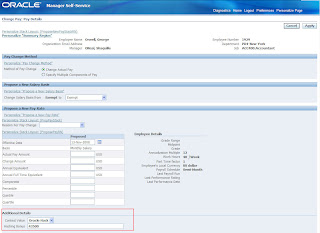
For extra credit and part II of this topic, you can set the DFF segment to Required in the CO as well. Here is the code; note that the "yes" text is case-sensitive:


In 11.5.10, this seemed to not be possible, at least not in my experience hacking at it. Note that setting a DFF segment to required in the Controller is different from setting up a DFF segment as required when it is configured. When a segment is set to required in the application, it is required on all responsibilities throughout the app. Using the custom code approach allows you to mark specific segments required only when necessary.